|
 |
CS 3723/3721
Programming Languages
Homework
|
|
1. Return Address:
You are to write a Postscript program that will create the
return address and "postage paid" announcement exactly
as shown below.
Return Address for a mailing |
 |
Click on the image to get a PDF file
You should include the following features:
- the two fields shown must be exactly 0.5 inch
(36 points) from the top of an 8.5-by-11 inch sheet of paper,
- margins must be exactly 0.5 inch (36 points).
- The font on the left should be given by
Times-Bold findfont 14 scalefont setfont
- The font on the right should be the same, except 12 point
- all linewidths should be given by 2 setlinewidth.
- The length of the lines at the left should be the same as
(The University of Texas at San Antonio) stringwidth pop,
that is, by the length of this string.
- lines on the left should be 15 points apart vertically.
- lines on the right should be 12 points apart vertically.
- The box on the right should be 132 points wide.
- Each line of the box on the right should be exactly centered
in the middle of the box. (See the page
Right Justifying Text,
which also mentions centering text, a similar task.)
The image above is an actual image, 8.5 inches wide, taken from
a program that met the requirements above.
2. A Knot:
You are to write a single Postscript program that will draw versions of
a simple knot, including the 3 below.
Three versions of a Postscript Knot Program |
x: 4, vertices labeled | x: 8 | x: 12 |
 |
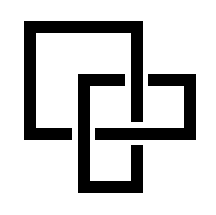 |
 |
You should make x a variable inside the program.
You should draw the three line segments
that make up the "knot" with linewidth 2*x, and allow a "gap"
at either end of each line segment of width x.
(The end of each line segment is then a distance of 2*x
from the center of the other line segments.)
The numbers in the left-hand picture give the coordinates of each corner of the
paths used. Notice that these are just whole "inches".
You can then get the three pictures above by taking x
equal to the value above each picture.
(Of course without the numbers in the left-hand picture.)
What will the picture look like when x has
value 24?
3. Circles: This part is concerned
with drawing circles.
To draw circle with center coordinates
x and y, and with radius r you can use
the following (see the Bluebook, pages 53 to 60):
newpath x y r 0 360 arc stroke
You are to write a Postscript program that will draw an array
of n circles, each of radius
r and the centers of these circles
should be arranged around a central point in
a circle of radius s. These parameters
should be variables in your program:
- n: the number of circles
- r: the radius of each circle
- s: the radius of the circle giving the centers
of the circles.
The pictures below give a little more information: In the last 4
pictures the first
circle is drawn wider and in red.
The circle of the centers of circles is drawn in
"CornflowerBlue".
3: Circles |
n:10, r:100, s:100 | n: 20, r:100, s:100 | n: 40, r:100, s:100 |
 |
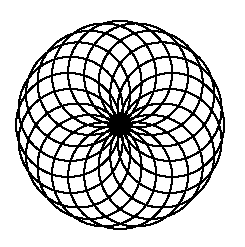 |
 |
n:40, r:50, s:150 | n: 20, r:150, s:50 | n: 30, r:110, s:90 |
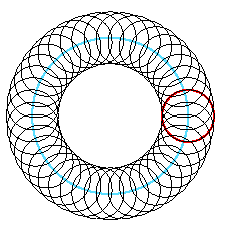 |
 |
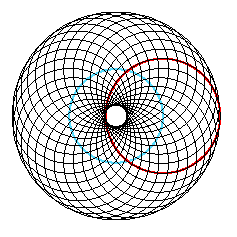 |
Implementation: There are several simple ways to program
this in Postscript. You should center everything at the origin,
translate to the center of the page, and draw everything.
You can let an angle th take on the necessary
successive values. Then the center of each circle of radius r will
be at the point (s*cos(th), s*sin(th), and you can draw each
circle.
Alternatively, you can draw one circle and keep rotating by
the successive angle: 360/n.
4. Stars:
You are to write a Postscript program that will produce any
of a variety of "stars", similar to the ones given below:
| |
|
Click Image for .pdf | |
Click Image for .pdf |
Detailed Requirements:
Your program should conform the the following requirements:
- You should create the star centered at the origin.
- You can then translate the center of the star to
wherever you want it before rendering it. (In my pictures I
did all 6 at once.)
- The radius of the star should be exactly 100 points.
- The star should should be drawn with a function (perhaps
named star), that uses two input parameters on the Postscript stack:
n, the number of star "tips", and
m, the number of tips to skip ahead in
drawing a line. Thus the six stars above have parameters
(left-to-right and top-to-bottom)
5 2, 10 3,
20 6, 20 9,
12 4, and 18 6.
In the second star, with parameters 10 and 3, there are
10 tips, and you draw a line from each tip to the tip that is
3 ahead in the sequence of tips.
In your program, the second star might be called with code
like: 10 3 star.
- You must use a for loop (or some other loop), so that your program
will work for any reasonable input.
(You don't need code to verify "reasonable input".)
- If you use code that you get from some other source
(wherever it might be from: the web, textbooks, my own materials,
or another student),
then you must cite this source in the code that you submit.
Hints and Suggestions:
Here are a few hints:
- There are many ways to complete this assignment.
- Perhaps the simplest method is to draw
each line, one at a time. (This approach was used in the image
at the left above. It works well, but it ends up with flat
star "tips".)
- Alternatively, you could try to mimic the star program presented
in the course and in the text. That approach is tricky,
and does the star as a single path or as several paths.
(With this more difficult approach, the star tips are pointed.)
- You could calculate the tips of the star, using simple equations,
so that (100, 0) can be one tip, and locations of the others depend on
how many there are (divide 360 by n to get
the angle between tips), and then use simple formulas
(involving sin and cos) for the locations of other tips.
- After drawing one line, one simple method (but by no means the
only one), is to use rotate by the angle between tips
to get the other lines.
- Notice that stars 3, 5, and 6 above are different from the other
3 in a significant way. (The red line is a hint about this difference.)
For some ways of drawing the stars, this difference won't matter,
while for others it will.
- Any use of translate or rotate
(or any other change of the graphics state)
enclosed inside the Postscript commands
gsave and grestore will not have an
effect outside these enclosed commands. So you can do a
rotate after gsave, do some
other stuff, and then after a grestore
the effect of the rotate is reversed. This use of
gsave and grestore is illustrated
and explained at the end of the "star" example:
Star
5. Business Card:
For this recitation, you are to write a program in Postscript that
will print a "business card", either for you, or for some fictitious
individual or company. Graphic art often uses a principle of
"less is more", meaning that a simple design is often more
satisfactory than a more complex one. Here are some examples,
some simple, some not so simple.
However, this is not an art assignment, and you are not being graded
on "good graphic design." Instead I want you to experiment with
complicated Postscript code to help learn the language.
Here are items I want you to include in the recitation:
- The initial card should be exactly
4 inches by 7 inches.
(That is, the card should be exactly 288 points by 504 points.)
- The outer 4 inch by 7 inch boundary should be stroked with a
line of width 3 points.
- With one run, translating the card if necessary, print a
single copy of your card on a sheet of paper.
Again, this should be 4 inches by 7 inches.
- In a separate run, using a scale factor of 0.5 in each direction,
produce at least 5 copies of the same card at half size, that is,
of size 2 inches by 3.5 inches.
The Postscript
code that produces these 5 copies should employ a Postscript
loop. (See pages 51-52 and Chapter 7 of the
Blue Book (PDF, 847k).)
- At least one piece of text on the card should
either be centered
or right justified, using the stringwidth function, so that the
text would remain centered or right-justified even if the font
size is changed. (See the Blue Book, pages 42-43 for right justification,
and pages 58-59 for centering. See also my handout with a card on it.)
Here are some additional features that I would like to see you
incorporate into your card:
- The use of some ``graphic object'', e.g., some picture made up
of one or more paths. For example, a recursive structure like
that on pages 73-75 of the Blue Book would be interesting.
- Use some color, although your final printout doesn't
have to be on a color printer.
- The use of an outline around characters in a string. (See the
example on page 98 of the Blue Book.)
- The use of a clipping path. (See the Blue Book, pages 103-104.)
Finally, try to make it look interesting. Be creative.
The card doesn't have to have your real name. Don't
copy the book or one another or my cards below.
(Some students might submit, say, exactly the "Diamond Cafe"
card below with different words stuck it. This would be worth
little or no credit.)
Examples of Cards:
Two cards from the Blue Book plus one of my own:
( Revision date: 2014-04-13.
Use the ISO 8601
standard.)
|